Jun 03, 2019
Image credit
Hi there!
Thought I'd give a big hi today in apologies for the lack of length that this blog post has in comparison with my somewhat hectic previous one. I can't consistently keep banging out blog posts that long though, I'm pretty busy.
So, List Comprehensions
Moving right along I have learnt how to use a fantastic paradigm in python for creating lists based on other lists called list comprehensions.
Take the following:
A list of numbers
old_list = [1,2,3,4,5,6,7,8,9,10]
Create a new list
new_list = []
Iterate through previous list and add only the even numbers to the new list.
for number in old_list:
if number % 2 == 0:
new_list.append(number)
Cool! So obviously what we are trying to do here is extract the even numbers from the old list.
So lets try that out using list comprehensions:
A list of numbers
old_list = [1,2,3,4,5,6,7,8,9,10]
new_list = [number for number in old_list if number % 2 == 0]
Would you look at that? The new list creation is now a one liner!
Awesome, so how does it work?
Glad you asked. So, as the name suggests, python is capable of 'comprehending' lists in a simplified way that makes reading a breeze (once you get used to it). A basic format might be something like:
list = [ITEM FROM ORIGINAL LIST for ITEM FROM ORIGINAL LIST in ORIGINAL LIST]
Alternatively, you could swing in the opposite direction and just go to town on the conditions and chain if statements like so:
new_list = [number for number in old_list if number > 1 if number < 3]
result: [2]
That's all for this week!
Like I said, short and sweet today. Tune in next week for some more geek talk!
May 27, 2019
Image credit
Why A Star?
So for game development, we were in a situation where we had a player that you could swap for any one of three characters. These characters needed to follow along behind the main character.
At first, I thought I could do something simple like:
if(player.x > character.x) character.x++
if(player.x < character.x) character.x--
This is obviously a simplification but it illustrates the general idea. So then jumping becomes an issue. Say we have a hole in the ground in front of the player. The player jumps over it, leaving the following character in an existential crisis about how to overcome the dilemma before it.
You could have the player object leave some kind of marker indicating a jump needed to be performed here and there are indeed some pathfinding systems that work this way. Donkey Kong on the Super Nintendo did something like this with its character following.
This was not an option so much for us, however, as we planned to be able to use this same algorithm to have enemies find their way around complex maps.
After a considerable amount of research, we settled on the A Star algorithm. This algorithm basically finds the shortest available route between two points in a grid of nodes.
What are we working with here?
As you may remember from my previous post, I use a little piece of software called GameMaker Studio 2. It works pretty well and I have spent many hours coding in its GML language so I am aware mostly of its strengths and weaknesses.
GMS2 has this concept of objects in it which is great. You can create objects and within each one is housed all of its code that is ran for every loop of the game. Objects can also inherit from each other just like any other object-oriented language.
// Player object
// Basic movement.
speed = 10;
movement = keyboard_check(vk_right) - keyboard_check(vk_left); //Checks return boolean 0 or 1
x += movement * speed;
The above code here runs inside of the player objects step event. This runs every frame. This will either move the player forward or backward or not at all depending on what is pressed. Super easy right?
But at what cost?
The main cost is not being able to define your own classes. Building custom data structures can be a real pain. I read somewhere (sorry, i cant remember where) that the creators tacked on some functions that build pseudo-data structures after GameMaker became popular as it was originally intended as a simple learning language. Things like lists, maps (aka dictionaries), grids (dynamic 2d arrays), stacks, queues etc. were implemented. For the most part, these are sufficient to build some cool stuff.
But I want to build a network of nodes that I can store my own custom properties:
node = {
"x" : [],
"y" : [],
neighbours : [
{"relationship_type" : "", "node" : node}
]
};
These are just a few of the properties I need to store.
A Star gets a little complicated.
A star is pretty hectic.
It basically goes through a set of nodes and checks the distance from the origin in node hopes and the Manhatten distance to the destination while noting a whole bunch of information on the way. Once it arrives at the end, it goes back through the nodes it found and from this data deduces the shortest path.
So what we had to do first was create a grid that divided the whole playable gaming area up into squares. Then we had to write some fairly complicated code to determine which of these squares was actually traversable.
It doesn't end there. We next needed to calculate the relationship between all these nodes based on the rules of the system. How high can you jump? How fast can you walk? This took a special amount of time to deduce.
Once we had all of that, we could plug in our A Star and it would give us a path. We simply tell a sprite to follow the coordinates it output and bam! You have a working AI traversal system.
What I've learned
Unfortunately, as I had no access to custom classes, I was forced to use a horrible mish-mash of maps and grids to store this data. This caused an unnecessary gap between my mind as a developer and what the computer is actually doing as a lot of this code is incomprehensible without a few hours to recap and some decent documentation.
Basically, GMS2 may be more limited than I had initially anticipated.
I do love it for its simplicity but it is exactly that which causes these kind of problems. I'd imagine had I had the ability to make classes, I would have created node and path classes; each with some super awesome methods and properties to make this puzzle far better abstracted.
In future, we may consider using Unity.
What now?
One thing this experience makes me want to do is record a video tutorial on how to set A Star up in GMS2 as we put a lot of effort into it and resources were limited for us. I may post a link to that in here in the coming months. Finding the time could be difficult but I really would like to do it.
At this stage, we are going to put this project on hold and make a smaller title using GMS2. I think this is a good decision for the immediate future for us.
May 21, 2019
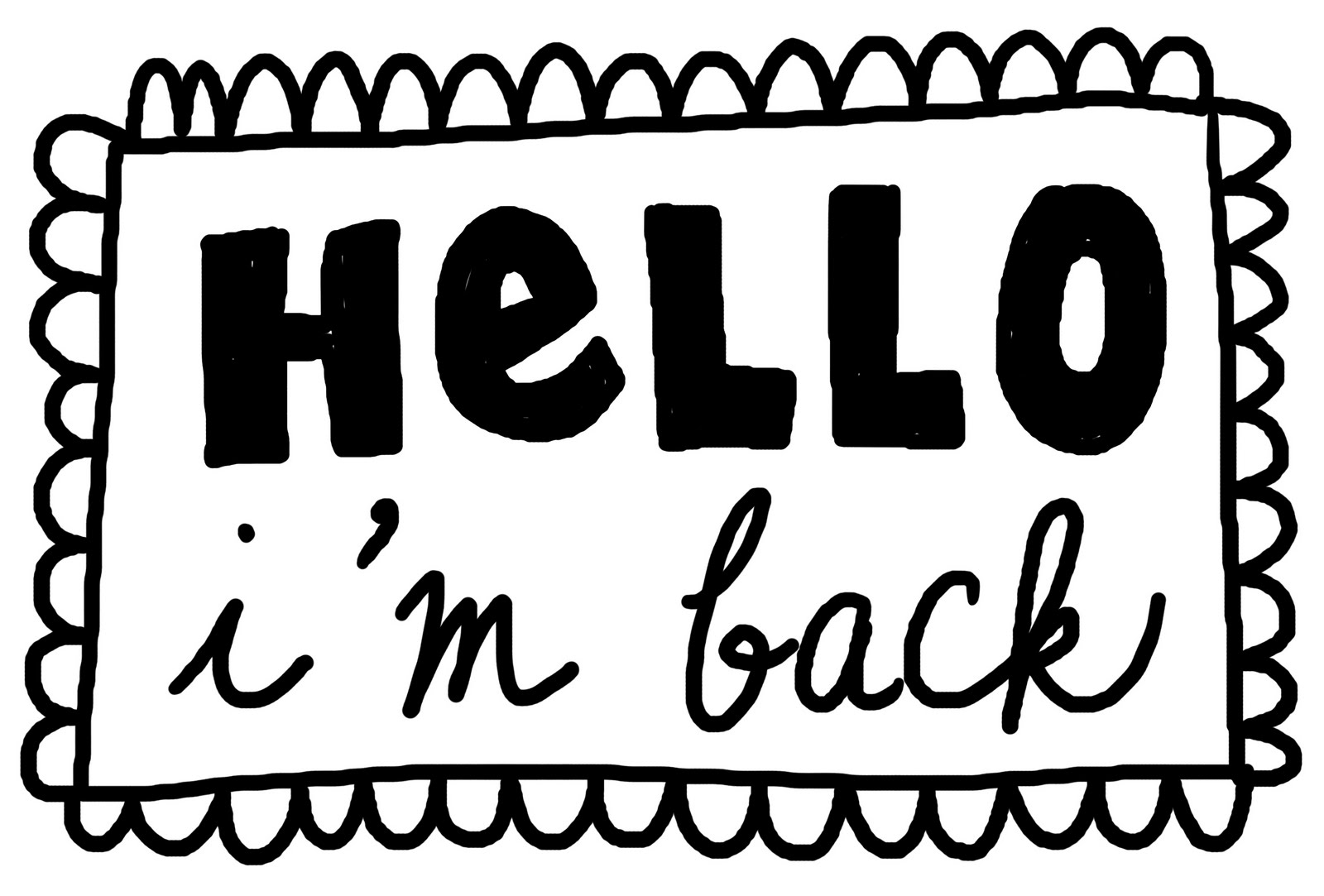
Image credit
Hello friends.
So it's been a while and I apologise. I haven't stopped though, I have just been busy getting things done.
So what's been happening? That was more of a rhetorical question as you are reading and I have hopefully moved on since writing this. I've been working. A lot.
University
I'm still completing my university degree with one more semester after the current to go. This year, I am studying Algorithms & Complexity which of course is a super interesting subject, though if I'm honest, is being communicated poorly. The lecturer just reads walls of text off a screen and shows working in a limited way which is not helpful to me. I usually just check the current subject he is discussing and use YouTube to learn it.
The other subject is the Capstone Project. In this subject, we are given an industry project by the university and told to do it over two semesters to give us experience in the real world. We were given a project requiring us to upgrade a previous years project. This was a board that special education students could interact with ina way that compliments their particular disability.
We are in the process of finalising our plans. We decided to upgrade the hardware in the board to make it function independently. We are using a Raspberry Pi, Python and the library PyGame to create something interactive that is capable of switching on and off different output devices. So far, the unit has been quite rewarding and I am absolutely invested in the final product.
Game Development
So this has been getting a little difficult to squeeze in given the volume of responsibilities I have been investing myself in. But as slow as it may be, I am passionate about these side projects.
Since I last posted in here, I completed to mini 'for laughs' project and have moved on to creating something else. I got myself a couple of partners and started a business, AntiType. We are a group of three: an Artist, a Writer and myself (the coder). We were working on an up and coming platformer called For Loot & Glory (based on our writers trilogy). But alas, it was too ambitious. That project is on hold until we get some experience.
We are now in the process of fleshing out a new concept game that will be available at the end of the year. We are playing this one pretty close to our chest so watch this space!
Band
The news on this front is not great. Unfortunately, we all decided to part ways. My reason for doing so was I had simply bitten off more than I could chew.
While I hope this is in my future again, for now, I am a former rockstar.
Work
This blog turned out to be useful for getting work. At the beginning of 2018, I got a job at a company called Colourwise. We specialise in direct marketing and I am responsible for developing automated systems that run regular jobs and building templates for ad hoc work. It's fantastic. So fantastic, that I forgot to post on here for over a year!
In my new occupation, I have been getting very familiar with the Python programming language. I have had the privilege of building from scratch a library for interacting with our printing software more efficiently which are giving me an ever-deepening understanding of Python. I find it fascinating and am so grateful for the many opportunities I have been given to do so.
Conclusion
Sorry this was such a long post. I just wanted to catch up on a few items before getting into the more concise stuff. Next week, I might show some of the things I have been doing over the last year in a bit more detail.
Until then, stay classy!